CODING ASSIGNMENT 3 Library Management
Library Management
In this assignment, let's build a Library Management page by applying the concepts we learned till now.
Refer to the image below
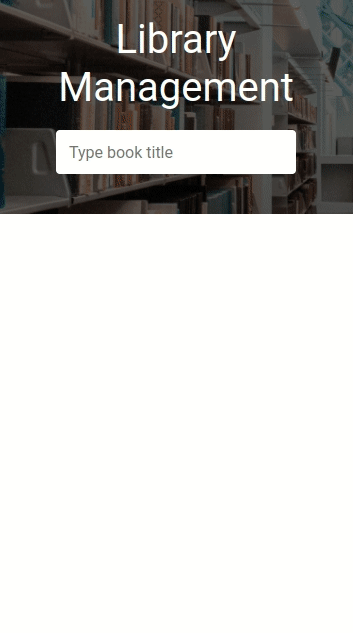
Completion Instructions
Instructions
Functionality to be added
HTTP Requests & Responses
Resources
Colors
Font-families
Concepts Review
Want to quickly review some of the concepts you’ve been learning?
Take a look at the Cheat Sheets.
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous" /> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.1/dist/umd/popper.min.js" integrity="sha384-9/reFTGAW83EW2RDu2S0VKaIzap3H66lZH81PoYlFhbGU+6BZp6G7niu735Sk7lN" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script> </head> <body> <div class="container"> <div class="row"> <div class="p-4 col-12 searchContainer"> <h1 class="mainHeading">Library Management</h1> <input class="w-90" placeholder="Type book title" type="search" id="searchInput" /> </div> <div class="col-12"> <div class="mt-3 text-center"> <div id="spinner" class="d-none spinner-border text-dark" role="status"> <span class="sr-only">Loading...</span> </div> <p id="message"></p> </div> </div> <div id="searchResults" class="col-12"> </div> </div> </div> </body> </html>
@import url("https://fonts.googleapis.com/css2?family=Bree+Serif&family=Caveat:wght@400;700&family=Lobster&family=Monoton&family=Open+Sans:ital,wght@0,400;0,700;1,400;1,700&family=Playfair+Display+SC:ital,wght@0,400;0,700;1,700&family=Playfair+Display:ital,wght@0,400;0,700;1,700&family=Roboto:ital,wght@0,400;0,700;1,400;1,700&family=Source+Sans+Pro:ital,wght@0,400;0,700;1,700&family=Work+Sans:ital,wght@0,400;0,700;1,700&display=swap");
.searchContainer {
background-image: url('https://assets.ccbp.in/frontend/dynamic-webapps/library-management-bg.png');
width: 100%;
background-size: cover;
text-align: center;
}
.mainHeading {
color: #ffffff;
}
let searchInput = document.getElementById("searchInput");
let searchResults = document.getElementById("searchResults");
let message = document.getElementById("message");
let headingEl = document.createElement("h1");
let spinner = document.getElementById("spinner");
function appendSearchResults(search_results) {
if (search_results.length < 1) {
message.textContent = "No results found";
searchResults.textContent = "";
headingEl.textContent = "";
} else {
searchResults.textContent = "";
message.textContent = "";
headingEl.textContent = "Popular Books";
searchResults.appendChild(headingEl);
for (let eachItem of search_results) {
let title = eachItem.title;
let image = eachItem.imageLink;
let author = eachItem.author;
let imageEl = document.createElement("img");
let textEl = document.createElement("p")
imageEl.setAttribute("src", image);
textEl.textContent = author;
searchResults.appendChild(imageEl);
searchResults.appendChild(textEl);
console.log(eachItem);
}
}
}
searchInput.addEventListener("keydown", function(event) {
if (event.key === "Enter") {
spinner.classList.toggle("d-none");
let searchInputValue = searchInput.value;
let url = "https://apis.ccbp.in/book-store?title=" + searchInputValue;
let options = {
method: "GET"
};
fetch(url, options)
.then(function(response) {
return response.json();
})
.then(function(jsonData) {
let {
search_results
} = jsonData;
appendSearchResults(search_results);
spinner.classList.toggle("d-none");
});
}
});
Comments
Post a Comment