CODING ASSIGNMENT 3 Speed Typing Test
Speed Typing Test
In this assignment, let's build a Speed Typing Test by applying the concepts we learned till now.
Refer to the below image.
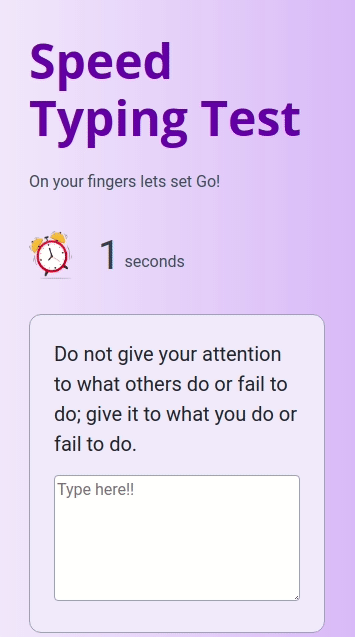
Instructions:
- Add HTML container element with id speedTypingTest
- Add HTML paragraph elements with id timer,quoteDisplayandresult
- Add HTML textarea element with id quoteInput
- Add HTML button elements with id submitBtnandresetBtn
- Add the Bootstrap component spinner
By following the above instructions, achieve the given functionality.
- When the page is opened
- Make a HTTP request to get a random quotation
- URL: https://apis.ccbp.in/random-quote
- URL:
- Display the random quotation in the HTML paragraph element with id quoteDisplay
- Start the timer and display the time in seconds in the HTML paragraph element with id timer
- Make a HTTP request to get a random quotation
- When a value is entered in the HTML textarea element with id quoteInputand the HTML button element with idsubmitBtnis clicked
- If the value entered in the HTML textarea element with id quoteInputis same as the quotation in the HTML paragraph element with idquoteDisplay
- The timer should be stopped and a success message should be shown in the HTML paragraph element with id result
- The timer should be stopped and a success message should be shown in the HTML paragraph element with id
- If the value entered in the HTML textarea element with id quoteInputdoes not match the quotation in the HTML paragraph element with idquoteDisplay
- The timer should be running and an error message should be shown in the HTML paragraph element with id result
- The timer should be running and an error message should be shown in the HTML paragraph element with id
- If the value entered in the HTML textarea element with id
- When the HTML button with id resetBtnis clicked
- Make a HTTP request to get a new random quotation
- Display the new random quotation in the HTML paragraph element with id quoteDisplay
- Reset the timer to 0seconds and display the time in seconds in the HTML paragraph element with idtimer
- Empty the HTML textarea element with id quoteInput
- Add loadingstatus with Bootstrap componentspinnerwhile making an HTTP request.
Resources
Clock Image:
CSS Colors Used:
Background Color Hex Codes Used:
Border Color Hex Codes Used:
Text Color Hex Codes Used:
Concepts Review
Want to quickly review some of the concepts you’ve been learning?
Take a look at the Cheat Sheets.
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous" /> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.1/dist/umd/popper.min.js" integrity="sha384-9/reFTGAW83EW2RDu2S0VKaIzap3H66lZH81PoYlFhbGU+6BZp6G7niu735Sk7lN" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script> </head> <body> <div class="container p-3"> <div class="row"> <div id="speedTypingTest" class="col-12"> <h1>Speed Typing test</h1> <p>On your fingers lets set Go!</p> <div class="d-flex flex-row"> <img class="mr-3" src="https://assets.ccbp.in/frontend/dynamic-webapps/clock-img.png " alt="" /> <p id="timer">0</p> <p class=" ml-2 mt-4">seconds</p> </div> </div> <div class="col-12"> <div class="cardContainer"> <p id="quoteDisplay"></p> <textarea placeholder="Type Here" class="form-control" id="quoteInput" rows="5" cols="20"></textarea> </div> <div class="text-center text-light mt-3"> <div id="spinner" class="d-none spinner-border" role="status"> <span class="sr-only">Loading...</span> </div> </div> <p id="result"></p> <div class="text-center"> <button class="btn" id="submitBtn" type="submit">Submit</button> <button class="btn ml-2" id="resetBtn" type="submit">Reset</button> </div> </div> </div> </div> </body> </html>
@import url("https://fonts.googleapis.com/css2?family=Bree+Serif&family=Caveat:wght@400;700&family=Lobster&family=Monoton&family=Open+Sans:ital,wght@0,400;0,700;1,400;1,700&family=Playfair+Display+SC:ital,wght@0,400;0,700;1,700&family=Playfair+Display:ital,wght@0,400;0,700;1,700&family=Roboto:ital,wght@0,400;0,700;1,400;1,700&family=Source+Sans+Pro:ital,wght@0,400;0,700;1,700&family=Work+Sans:ital,wght@0,400;0,700;1,700&display=swap"); .container { background-color: #dac0ff; height: auto; } .cardContainer { background-color: #f3eaff; height: auto; width: auto; padding: 20px; border-radius: 20px; border: 2px solid #9aa5b1; } h1 { color: #690cb0; } #submitBtn { background-color: #690cb0; color: white; } #resetBtn { background-color: #f2ebf3; } img { height: 60px; } span { font-size: 16px; } #timer { font-size: 40px; }
let timer = document.getElementById("timer");
let quoteDisplay = document.getElementById("quoteDisplay");
let result = document.getElementById("result");
let quoteInput = document.getElementById("quoteInput");
let submitBtn = document.getElementById("submitBtn");
let resetBtn = document.getElementById("resetBtn");
let spinner = document.getElementById("spinner");
let counter = 0;
spinner.classList.toggle("d-none");
function startCounter() {
counter += 1;
timer.textContent = counter;
console.log(counter);
}
let counterValue = setInterval(startCounter, 1000);
function getQuote() {
let options = {
method: "GET"
};
fetch("https://apis.ccbp.in/random-quote", options)
.then(function(response) {
return response.json();
})
.then(function(jsonData) {
spinner.classList.add("d-none");
let quote = jsonData.content;
quoteDisplay.textContent = quote;
console.log(jsonData.content);
});
}
getQuote();
startCounter();
resetBtn.onclick = function() {
spinner.classList.remove("d-none");
getQuote();
startCounter();
counter = 0;
result.textContent = "";
quoteInput.value = "";
};
submitBtn.onclick = function() {
if (quoteInput.value === quoteDisplay.textContent) {
clearInterval(counterValue);
result.textContent = "You Typed in " + counter + " seconds";
} else {
result.textContent = "You typed Incorrect Sentence";
}
};
Comments
Post a Comment