CODING PRACTICE 1 Color Picker
Color Picker
In this assignment, let's build a Color Picker by applying the concepts we learned till now.
Refer to the below image.
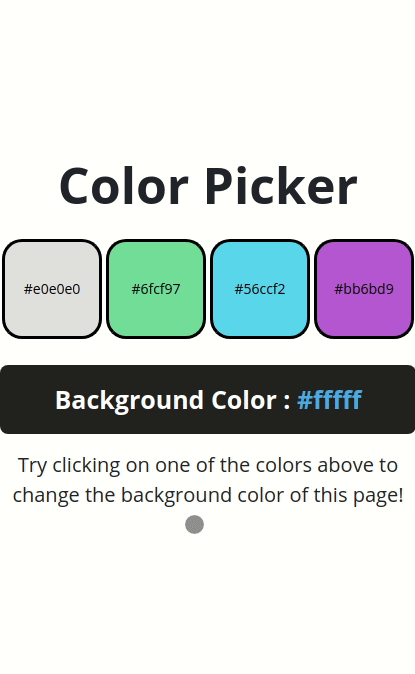
Instructions:
- The HTML container element that is wrapping all the HTML elements should have the id colorPickerContainer
- The HTML spanelement should have the idselectedColorHexCode
- The HTML buttonelement with text as #e0e0e0 should have the idbutton1
- The HTML buttonelement with text as #6fcf97 should have the idbutton2
- The HTML buttonelement with text as #56ccf2 should have the idbutton3
- The HTML buttonelement with text as #bb6bd9 should have the idbutton4
By following the above instructions, achieve the given functionality.
- The background color of the page and the Hex Code value in the HTML spanelement should change when the HTMLbuttonelement is clicked.
- The background color of the page and the background color of the clicked HTML buttonelement should be the same.
- The text in the clicked HTML buttonelement and the text in the HTMLspanelement should be the same.
- Try to achieve the design as close as possible.
- Apply the styles and functionality to the given HTML prefilled code.
Resources
CSS Colors used:
CSS Font families used:
- Open Sans
Concepts Review
Want to quickly review some of the concepts you’ve been learning?
Take a look at the Cheat Sheets.
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous" /> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.1/dist/umd/popper.min.js" integrity="sha384-9/reFTGAW83EW2RDu2S0VKaIzap3H66lZH81PoYlFhbGU+6BZp6G7niu735Sk7lN" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script> </head> <body> <div id="colorPickerContainer" class="d-flex flex-column justify-content-center"> <h1>Color Picker</h1> <div> <button class="button btn1" onclick="colorOne()" id="button1">#e0e0e0</button> <button class="button btn2" onclick="colortwo()" id="button2">#6fcf97</button> <button class="button btn3" onclick="colorthree()" id="button3">#56ccf2</button> <button class="button btn4" onclick="colorfour()" id="button4">#bb6bd9</button> </div> <p class="Background_Color mt-3 mb-3">Background Color : <span id="selectedColorHexCode">#fffff</span></p> <p>Try clicking on one of the colors above to change the background color of this page!</p> </div> </body> </html>
@import url('https://fonts.googleapis.com/css2?family=Bree+Serif&family=Caveat:wght@400;700&family=Lobster&family=Monoton&family=Open+Sans:ital,wght@0,400;0,700;1,400;1,700&family=Playfair+Display+SC:ital,wght@0,400;0,700;1,700&family=Playfair+Display:ital,wght@0,400;0,700;1,700&family=Roboto:ital,wght@0,400;0,700;1,400;1,700&family=Source+Sans+Pro:ital,wght@0,400;0,700;1,700&family=Work+Sans:ital,wght@0,400;0,700;1,700&display=swap');
#colorPickerContainer {
height: 100vh;
text-align: center;
}
h1 {
font-weight: 900;
font-size: 35px;
margin-bottom: 20px;
}
.button {
height: 90px;
width: 90px;
border-radius: 20px;
border-style: solid;
border-width: 2px;
font-size: 12px;
}
.btn1 {
background-color: #e0e0e0;
}
.btn2 {
background-color: #6fcf97;
}
.btn3 {
background-color: #56ccf2;
}
.btn4 {
background-color: #bb6bd9;
}
.Background_Color {
background-color: #222222;
color: #ffffff;
padding: 10px;
font-size: 22px;
font-weight: 600;
border-radius: 10px;
}
#selectedColorHexCode {
color: #49a6e9;
}
let colorPickerContainer = document.getElementById("colorPickerContainer");
let selectedColorHexCode = document.getElementById("selectedColorHexCode");
function colorOne() {
colorPickerContainer.style.backgroundColor = "#e0e0e0";
selectedColorHexCode.textContent = "#e0e0e0";
}
function colortwo() {
colorPickerContainer.style.backgroundColor = "#6fcf97";
selectedColorHexCode.textContent = "#6fcf97";
}
function colorthree() {
colorPickerContainer.style.backgroundColor = "#56ccf2";
selectedColorHexCode.textContent = "#56ccf2";
}
function colorfour() {
colorPickerContainer.style.backgroundColor = "#bb6bd9";
selectedColorHexCode.textContent = "#bb6bd9";
}
Comments
Post a Comment