CODING PRACTICE 1 Traffic Light
Traffic Light
In this assignment, let's build a Traffic Light by applying the concepts we learned till now.
Refer to the below image.
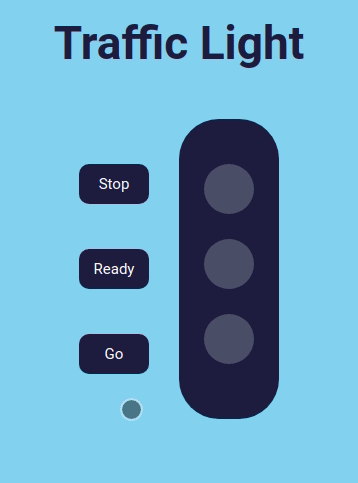
Instructions:
- The HTML buttonelements should have the idsstopButton,readyButton, andgoButton
- The HTML container elements should have the ids stopLight,readyLight, andgoLight
By following the above instructions, achieve the given functionality.
- When stopButtonis clicked, the background color of thestopLightcontainer and thestopButtonelement should change to red. The other HTML elements should have their default background colors as shown in the image.
- When readyButtonis clicked, the background color of thereadyLightcontainer and thereadyButtonelement should change to yellow. The other HTML elements should have their default background colors as shown in the image.
- When goButtonis clicked, the background color of thegoLightcontainer and thegoButtonelement should change to green. The other HTML elements should have their default background colors as shown in the image.
- Try to achieve the design as close as possible.
- Apply the styles and functionality to the given HTML prefilled code.
- All the buttons should have the same background color by default.
- All the lights should have the same background color by default.
Resources
CSS Colors used:
CSS Font families used:
- Roboto
Concepts Review
Want to quickly review some of the concepts you’ve been learning?
Take a look at the Cheat Sheets.
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous" /> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.1/dist/umd/popper.min.js" integrity="sha384-9/reFTGAW83EW2RDu2S0VKaIzap3H66lZH81PoYlFhbGU+6BZp6G7niu735Sk7lN" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script> </head> <body> <div class="container"> <div class="row"> <div class="col-12 mt-3 mb-5"> <h1>Traffic Light</h1> </div> <div class="col-6 mt-3"> <div class="d-flex flex-column"> <button onclick="colorRed()" id="stopButton">Stop</button> <button onclick="colorYellow()" id="readyButton">Ready</button> <button onclick="colorBlue()" id="goButton">Go</button> </div> <div> <div></div> <div></div> <div></div> </div> </div> <div class="col-6"> <div class="light_box d-flex flex-column justify-content-center"> <button id="stopLight" class="button" type="submit"></button> <button id="readyLight" class="button" type="submit"></button> <button id="goLight" class="button" type="submit"></button> </div> </div> </div> </div> </body> </html>
@import url('https://fonts.googleapis.com/css2?family=Bree+Serif&family=Caveat:wght@400;700&family=Lobster&family=Monoton&family=Open+Sans:ital,wght@0,400;0,700;1,400;1,700&family=Playfair+Display+SC:ital,wght@0,400;0,700;1,700&family=Playfair+Display:ital,wght@0,400;0,700;1,700&family=Roboto:ital,wght@0,400;0,700;1,400;1,700&family=Source+Sans+Pro:ital,wght@0,400;0,700;1,700&family=Work+Sans:ital,wght@0,400;0,700;1,700&display=swap');
.container {
background-color: #86d2f2;
height: 100vh;
}
h1 {
text-align: center;
font-size: 40px;
font-weight: bold;
}
.button {
background-color: #4b5069;
width: 50px;
height: 50px;
border-radius: 40px;
margin: 5px;
}
#stopButton {
background-color: #1f1d41;
color: #ffffff;
border-radius: 10px;
width: 90px;
padding: 5px;
margin: 20px;
}
#readyButton {
background-color: #1f1d41;
color: #ffffff;
border-radius: 10px;
width: 90px;
padding: 5px;
margin: 20px;
}
#goButton {
background-color: #1f1d41;
color: #ffffff;
border-radius: 10px;
width: 90px;
padding: 5px;
margin: 20px;
}
.light_box {
height: 280px;
width: 100px;
border-radius: 40px;
background-color: #1f1d41;
padding: 20px;
}
let stopLight = document.getElementById('stopLight');
let readyLight = document.getElementById('readyLight');
let goLight = document.getElementById('goLight');
let stopButton = document.getElementById('stopButton');
let readyButton = document.getElementById('readyButton');
let goButton = document.getElementById('goButton');
function colorRed() {
stopButton.style.backgroundColor = "#cf1124";
readyButton.style.backgroundColor = "#1f1d41";
goButton.style.backgroundColor = "#1f1d41";
stopLight.style.backgroundColor = "#cf1124";
goLight.style.backgroundColor = "#4b5069";
readyLight.style.backgroundColor = "#4b5069";
}
function colorYellow() {
readyButton.style.backgroundColor = "#f7c948";
stopButton.style.backgroundColor = "#1f1d41";
goButton.style.backgroundColor = "#1f1d41";
readyLight.style.backgroundColor = "#f7c948";
goLight.style.backgroundColor = "#4b5069";
stopLight.style.backgroundColor = "#4b5069";
}
function colorBlue() {
goButton.style.backgroundColor = "#86d2f2";
readyButton.style.backgroundColor = "#1f1d41";
stopButton.style.backgroundColor = "#1f1d41";
goLight.style.backgroundColor = "#86d2f2";
stopLight.style.backgroundColor = "#4b5069";
readyLight.style.backgroundColor = "#4b5069";
}
Comments
Post a Comment